Published on May 26, 2020
TypeScript is a Dev Tool; Not Your Test Suite
This is not another article shitting on TypeScript, but trying to show what it offers and what it doesn't.
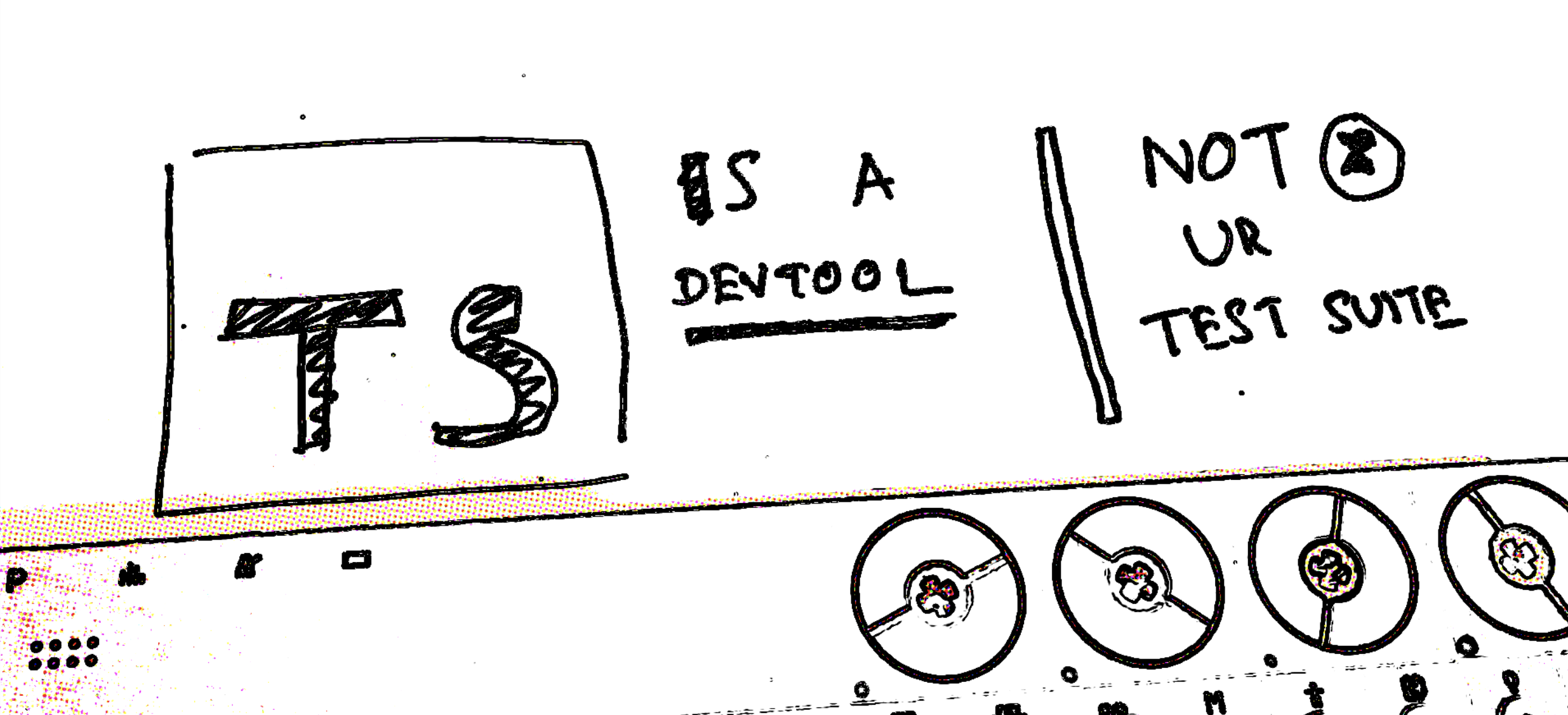
Sometimes, developers(and managers) think that using TypeScript will fix bugs or reduce potential bugs. In reality, TypeScript has very little to do with bugs, but it gives you these benefits (among others):
- Reduce human errors (This will prevent some bugs for sure)
- Faster development with code intelligence
- A form of documentation due to the use of types
So, what's missing?
It doesn't protect you from runtime errors. Let me share an example:
interface Payload {
email: string
transaction: number
}
interface HTTPRequest {
body: string
}
interface HTTPResponse {
send: (output: string) => void
}
function handleRequest(req: HTTPRequest, res: HTTPResponse) {
const payload = JSON.parse(req.body) as Payload
const currentBalance = 5000 - payload.transaction
const {email} = payload
res.send(JSON.stringify({ email, currentBalance }))
}
Q: What's the issue with this code block?
( Click to answer )
Another Example
interface ExternalLib {
sum: (a: number, b: number) => number;
}
async function calculatePoints(lib: ExternalLib) {
const newPoints = lib.sum(10, 20);
return newPoints * 1.1;
}
In this code, we use a third-party library; even it is correctly typed, we are not confident that it will always return a number.
Q: These third-party libraries are written with Typescript. So, why do I still need to worry?
( Click to answer )
Likewise, I can give a ton of examples like this. TypeScript alone cannot handle any runtime errors or knows how to deal with them. It still our job to take care of them.
So, if you think a bit, TypeScript is an upgrade from ESLint.
Any Solutions?
Actually, the solutions are not new or specific to TypeScript. It's the usual best practices when making apps. You have to use input validations and write tests.
For input validation, you can try using io-ts. It adds runtime type checking capabilities to Typescript. For that, you have to change how you create types, but that's worth it.
If we talk about tests, I don't think there's anything specific to TypeScript. Just use any framework you like. You'll get notable results when you started to write end to end testing.
Find the Right Balance
Now we know, TypeScript is not a substitute for testing even though it uses types. TypeScript alone does not make your app bulletproof.
So, shall I use it with my projects?
Okay. That's a tough question.
TypeScript adds a build step to your app. If you already have a build step, TypeScript will slow it down. There's a learning curve as well.
So, converting all your existing projects to use TypeScript may not be a wise idea.
We use TypeScript at Vercel for new projects and new functionalities. It improves dev time productivity and self-document the codebase, and that's a win for us.
TypeScript is just another devtool. So, think before you decide.
Don't follow the crowd.
Don't follow the crowd.
Oh! I forgot to mention this.
Google for "CoffeeScript." Then maybe "Flow type."
Google for "CoffeeScript." Then maybe "Flow type."